👥 Groups
Manage WhatsApp groups with the API.
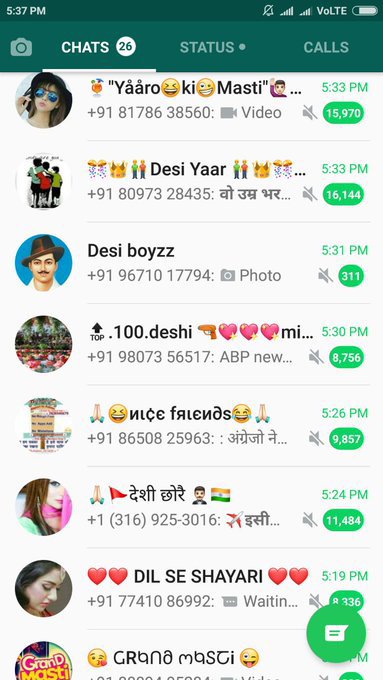
Features
Here’s the list of features that are available by 🏭 Engines:
👥 Groups - API
API | WEBJS | NOWEB | GOWS |
---|---|---|---|
POST /api/{session}/groups | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/count | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/join-info | ✔️ | ✔️ | ✔️ |
POST /api/{session}/groups/join | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/{id} | ✔️ | ✔️ | ✔️ |
DELETE /api/{session}/groups/{id} | ✔️ | ||
POST /api/{session}/groups/{id}/leave | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/{id}/picture | ✔️ | ✔️ | ✔️ |
PUT /api/{session}/groups/{id}/picture | ➕ | ➕ | ➕ |
DELETE /api/{session}/groups/{id}/picture | ➕ | ➕ | ➕ |
PUT /api/{session}/groups/{id}/description | ✔️ | ✔️ | ✔️ |
PUT /api/{session}/groups/{id}/subject | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/{id}/invite-code | ✔️ | ✔️ | ✔️ |
POST /api/{session}/groups/{id}/invite-code/revoke | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/{id}/settings/security/info-admin-only | ✔️ | ✔️ | ✔️ |
PUT /api/{session}/groups/{id}/settings/security/info-admin-only | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/{id}/settings/security/messages-admin-only | ✔️ | ✔️ | ✔️ |
PUT /api/{session}/groups/{id}/settings/security/messages-admin-only | ✔️ | ✔️ | ✔️ |
GET /api/{session}/groups/{id}/participants | ✔️ | ✔️ | ✔️ |
POST /api/{session}/groups/{id}/participants/add | ✔️ | ✔️ | ✔️ |
POST /api/{session}/groups/{id}/participants/remove | ✔️ | ✔️ | ✔️ |
POST /api/{session}/groups/{id}/admin/promote | ✔️ | ✔️ | ✔️ |
POST /api/{session}/groups/{id}/admin/demote | ✔️ | ✔️ | ✔️ |
- ➕ - Available in ➕ WAHA Plus
👥 Groups - Events
Events | WEBJS | NOWEB | GOWS |
---|---|---|---|
group.v2.join | ✔️ | ✔️ | ✔️ |
group.v2.leave | ✔️ | ✔️ | ✔️ |
group.v2.participants | ✔️ | ✔️ | ✔️ |
group.v2.update | ✔️ | ✔️ | ✔️ |
✔️ | ✔️ | ||
✔️ |
API
{session}
- use the session name for Whatsapp instance that you created withPOST /api/session
endpoint{groupId}
- group id in format123123123123@g.us
. You can get the id in a few ways:- By handling incoming message webhook.
- By getting all groups (see below).
- By creating a new group and saving the id.
Check 👤 Contacts - Lids if you see @lid
in participants list for a group
Create a new group
POST /api/{session}/groups
{
"name": "Group name",
"participants": [
{
"id": "123123123123@c.us"
}
]
}
Get all groups
GET /api/{session}/groups
Response: depends on 🏭 Engine you use.
Query parameters:
GET /api/{session}/groups?limit=10&offset=0&sortBy=subject&sortOrder=desc
If you see timeout or the request takes too long - consider using limit
parameter to get objects in smaller chunks
limit=10
- limit the number of chats to returnoffset=0
- skip the number of chats from the startsortBy={field}
- sort by fieldsortBy=id
- sort by group idsortBy=subject
- sort by group subject
sortOrder=desc|asc
- sort orderdesc
- descending order (New first, A-Z)asc
- ascending order (Old first, Z-A)
exclude=participants
- you can exclude participants data from the response
I see rate-overlimit in NOWEB
👉 If you seerate-overlimit
error with NOWEB engine - try enabling 🏭 NOWEB Store before using the endpoint!Get groups count
Get the total number of groups
GET /api/{session}/groups/count
{
"count": 10
}
Join group
If you have invite URL for a group (like https://chat.whatsapp.com/invitecode
), you can
POST /api/{session}/groups/join
{
"code": "invitecode"
}
or using full link:
{
"code": "https://chat.whatsapp.com/invitecode"
}
{
"id": "123123123@g.us"
}
Get join info for group
If you have invite URL for a group (like https://chat.whatsapp.com/invitecode
), you can get group info:
GET /api/{session}/groups/join-info?code=invitecode
or using full link (remember to encode the URL)
GET /api/{session}/groups/join-info?code=https%3A%2F%2Fchat.whatsapp.com%2Finvitecode
Response depends on engine you’re using
Refresh groups
If you see any inconsistency in groups list or in participants list, you can refresh the groups from the WhatsApp server:
POST /api/{session}/groups/refresh
⚠️ Do not call it frequently, it can lead to rate-overlimit
error. Usually groups API has all up-to-date information.
Get the group
GET /api/{session}/groups/{groupId}
Delete the group
DELETE /api/{session}/groups/{groupId}
Leave the group
POST /api/{session}/groups/{groupId}/leave
Group Picture
You can get, set and remove group picture
Get Group Picture
GET /api/{SESSION}/groups/{ID}/picture?refresh=false
{SESSION}
- session name{ID}
- group id. Remember to encode@
symbol -123123123123%40g.us
refresh=True
- force refresh the picture. By default, we cache it 24 hours. Do not frequently refresh the picture to avoidrate-overlimit
error.
{
"url": "https://example.com/picture.jpg"
}
url
can benull
if there’s no picture for the group
Set Group Picture
👉 Available in WAHA Plus version.
PUT /api/{SESSION}/groups/{ID}/picture
{SESSION}
- session name{ID}
- group id. Remember to encode@
symbol -123123123123%40g.us
{
"success": true
}
Delete Group Picture
👉 Available in WAHA Plus version.
DELETE /api/{SESSION}/groups/{ID}/picture
{SESSION}
- session name{ID}
- group id. Remember to encode@
symbol -123123123123%40g.us
{
"success": true
}
Set group subject
Updates the group subject.
Returns true
if the subject was properly updated. This can return false if the user does not have the necessary
permissions.
PUT /api/{session}/groups/{groupId}/subject
{
"subject": "Group name"
}
Set group description
Updates the group description.
Returns true
if the subject was properly updated. This can return false if the user does not have the necessary
permissions.
PUT /api/{session}/groups/{groupId}/description
{
"description": "Group description"
}
Security - update group info
Updates the group settings to only allow admins to edit group info (title, description, photo).
PUT /api/{session}/groups/{groupId}/settings/security/info-admin-only
{
"adminsOnly": true
}
Get the group settings to only allow admins to edit group info (title, description, photo).
GET /api/{session}/groups/{groupId}/settings/security/info-admin-only
{
"adminsOnly": true
}
Returns true
if the setting was properly updated. This can return false if the user does not have the necessary permissions.
Security - who can send messages
Updates the group settings to only allow admins to send messages.
PUT /api/{session}/groups/{groupId}/settings/security/messages-admin-only
{
"adminsOnly": true
}
Returns true
if the setting was properly updated. This can return false if the user does not have the necessary permissions.
Get the group settings to only allow admins to send messages.
GET /api/{session}/groups/{groupId}/settings/security/messages-admin-only
{
"adminsOnly": true
}
Participants
Get participants
GET /api/{session}/groups/{groupId}/participants
Add participants
POST /api/{session}/groups/{groupId}/participants/add
{
"participants": [
{
"id": "123123123123@c.us"
}
]
}
Remove participants
POST /api/{session}/groups/{groupId}/participants/remove
{
"participants": [
{
"id": "123123123123@c.us"
}
]
}
Admin
Promote to admin
Promote participants to admin users.
POST /api/{session}/groups/{groupId}/admin/promote
{
"participants": [
{
"id": "123123123123@c.us"
}
]
}
Demote to regular users
Demote participants by to regular users.
POST /api/{session}/groups/{groupId}/admin/demote
{
"participants": [
{
"id": "123123123123@c.us"
}
]
}
Invite code
Get invite code
GET /api/{session}/groups/{groupId}/invite-code
Then you can put it in the url https://chat.whatsapp.com/{inviteCode}
and send it to contacts.
Revoke invite code
Invalidates the current group invite code and generates a new one.
POST /api/{session}/groups/{groupId}/invite-code/revoke
Events
Read more about 🔄 Events.
👥 Groups - Events
Events | WEBJS | NOWEB | GOWS |
---|---|---|---|
group.v2.join | ✔️ | ✔️ | ✔️ |
group.v2.leave | ✔️ | ✔️ | ✔️ |
group.v2.participants | ✔️ | ✔️ | ✔️ |
group.v2.update | ✔️ | ✔️ | ✔️ |
✔️ | ✔️ | ||
✔️ |
group.v2.join
group.v2.join
happens when you join or are added to a group.
{
"event": "group.v2.join",
"session": "default",
"payload": {
"group": {
"id": "1231231232@g.us",
"subject": "Work Group",
"description": "Group description",
"invite": "https://chat.whatsapp.com/invitecode",
"membersCanAddNewMember": true,
"membersCanSendMessages": true,
"newMembersApprovalRequired": true,
"participants": [
{
"id": "99999@c.us",
"role": "participant"
}
]
},
"timestamp": 789456123,
"_data": {}
}
}
group
- group informationid
- group IDsubject
- group namedescription
- group descriptioninvite
- invite linkmembersCanAddNewMember
- if members can add new membersmembersCanSendMessages
- if members can send messagesnewMembersApprovalRequired
- if new members need approval from adminsparticipants
- list of participants (check group.v2.participants for more details)
timestamp
- event timestamp_data
- engine specific data
group.v2.leave
group.v2.leave
happens when you leave or are removed from a group.
{
"event": "group.v2.leave",
"session": "default",
"payload": {
"group": {
"id": "1231231232@g.us"
},
"timestamp": 789456123,
"_data": {}
}
}
group.id
- group IDtimestamp
- event timestamp_data
- engine specific data
group.v2.participants
group.v2.participants
happens when someone join or leave a group.
ℹ️ It might duplicate group.v2.join and group.v2.leave events for your ID.
{
"event": "group.v2.participants",
"session": "default",
"payload": {
"type": "join",
"timestamp": 1666943582,
"group": {
"id": "123456789@g.us"
},
"participants": [
{
"id": "123456789@c.us",
"role": "participant"
}
],
"_data": {}
}
}
type
- event type. Possible values:- join - when someone joins the group
- leave - when someone leaves the group
- promote - when someone is promoted to admin
- demote - when someone is demoted to regular participant
participants
- list of participants (contains only changed participants)id
- participant IDrole
- participant role. Possible values:- left - left the group
- participant - regular participant
- admin - group admin
- superadmin - group super admin
_data
- engine specific data
group.v2.update
group.v2.update
happens when the group information is updated.
{
"event": "group.v2.update",
"session": "default",
"payload": {
"group": {
"id": "1231231232@g.us",
"subject": "New Work Group Name"
},
"timestamp": 789456123,
"_data": {}
}
}
group
- group information with updates field (may contain all fields in some engines)- See details in group.v2.join
timestamp
- event timestamp_data
- engine specific data
group.join
⚠️ DEPRECATED. payload
has engine specific data. Use group.v2.leave instead.
{
"event": "group.join",
"session": "default",
"engine": "WEBJS",
"payload": {
...
}
}
group.leave
⚠️ DEPRECATED. payload
has engine specific data. Use group.v2.leave instead.
{
"event": "group.leave",
"session": "default",
"engine": "WEBJS",
"payload": {
...
}
}